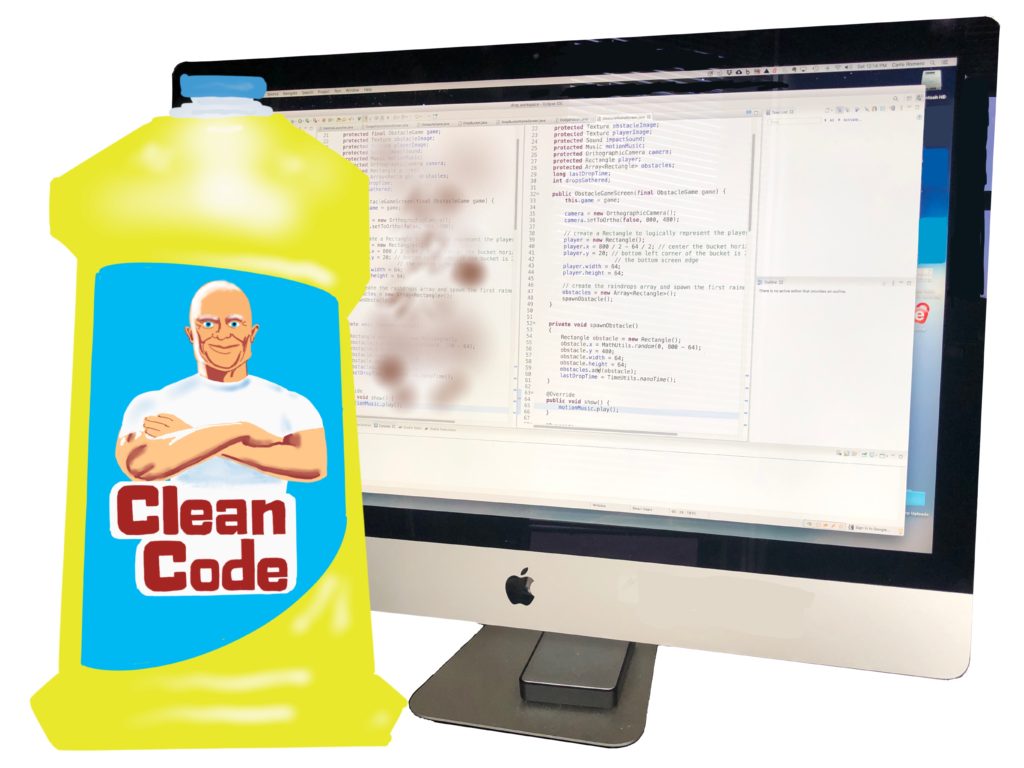
Introduction
- Software Development
- What is the point of running software?
- What should be your goal when you write code?
- What is the goal of a software project?
- A Software Product
- Software Design
- Why does code need to be designed?
- A design is a plan. Anything complex needs to be planned
- Any complex system needs to be designed before it is built
- If it’s not designed or designed well, this is what happens:
The Goals of Good Software Engineer:
- High quality software
- Measuring the Quality of Your Software: External Characteristics of Software Quality – What the Client Sees
- Correctness: The degree to which a system is free from faults in its specification, design, and implementation.
- Usability: The ease with which users can learn and use a system.
- Efficiency: Minimal use of system resources, including memory and execution time.
- Reliability: The ability of a system to perform its required functions under stated conditions whenever required—having a long mean time between failures.
- Integrity: The degree to which a system prevents unauthorized or improper access to its programs and its data. The idea of integrity includes restricting unauthorized user accesses as well as ensuring that data is accessed properly—that is, that tables with parallel data are modified in parallel, that date fields contain only valid dates, and so on.
- Adaptability: The extent to which a system can be used, without modification, in applications or environments other than those for which it was specifically designed.
- Accuracy: The degree to which a system, as built, is free from error, especially with respect to quantitative outputs. Accuracy differs from correctness; it is a determination of how well a system does the job it’s built for rather than whether it was built correctly.
- Robustness: The degree to which a system continues to function in the presence of invalid inputs or stressful environmental conditions.
- Measuring the Quality of Your Software: External Characteristics of Software Quality – What the Client Sees
- High quality code
- Measuring the Quality of your Code: Internal Software Quality Characteristics – What the Software Engineers Sees
- Maintainability: The ease with which you can modify a software system to change or add capabilities, improve performance, or correct defects.
- Flexibility: The extent to which you can modify a system for uses or environments other than those for which it was specifically designed.
- Portability: The ease with which you can modify a system to operate in an environment different from that for which it was specifically designed.
- Reusability: The extent to which and the ease with which you can use parts of a system in other systems.
- Readability: The ease with which you can read and understand the source code of a system, especially at the detailed-statement level.
- Testability: The degree to which you can unit-test and system-test a system; the degree to which you can verify that the system meets its requirements.
- Understandability: The ease with which you can comprehend a system at both the system-organizational and detailed-statement levels. Understandability has to do with the coherence of the system at a more general level than readability does.
- Measuring the Quality of your Code: Internal Software Quality Characteristics – What the Software Engineers Sees
- Quality Software is a function of high quality code
What is the difference between “software” and “code”?
- Software is the executing code
- “Application”, “App”, “Bot”, “Server” and so on are just different ways of “software”
- Why is it even called “software”?
- Why is it called “code”
Clean Code:
- From the book Clean Code
- Computers “understand” binary code, but human beings don’t
- Human beings communicate with language and tell stories.
- Code is not for the computers. It’s for you. It’s so you, the human being, the only thing in the universe that we know can think, can tell the computer what to do and get the expected results
- What is Clean Code?
- Bjarne Stroustrup, inventor of C++ and author of The C++ Programming Language:”I like my code to be elegant and efficient. The logic should be straightforward to make it hard for bugs to hide, the dependencies minimal to ease maintenance, error handling complete according to an articulated strategy, and performance close to optimal so as not to tempt people to make the code messy with unprincipled optimizations. Clean code does one thing well. Bad code tries to do too much, it has muddled intent and ambiguity of purpose. Clean code is focused. Each function, each class, each module exposes a single-minded attitude that remains entirely undistracted, and unpolluted, by the surrounding details.”
- Grady Booch, author of Object Oriented Analysis and Design with Applications: “Clean code is simple and direct. Clean code reads like well-written prose. Clean code never obscures the designer’s intent but rather is full of crisp abstractions and straightforward lines of control.”
- “Big” Dave Thomas, founder of OTI, godfather of the Eclipse strategy: “Clean code can be read, and enhanced by a developer other than its original author. It has unit and acceptance tests. It has meaningful names. It provides one way rather than many ways for doing one thing. It has minimal dependencies, which are explicitly defined, and provides a clear and minimal API. Code should be literate since depending on the language, not all necessary information can be expressed clearly in code alone. Code, without tests, is not clean. No matter how elegant it is, no matter how readable and accessible, if it hath not tests, it be unclean.”
- To summarize all of these:
- The code can be measured with either “good” or “bad” in the code review or by how many minutes it takes you to talk about it.
- A clean code should be elegant, efficient, readable, simple, without duplications, and well-written.
- You should add value to the business with your code.
- Clean code offers quality and understanding when we open a class. It is necessary that your code is clean and readable for anyone to find and easily understand. Avoid wasting others’ time.
- Clean Code is:
- Simple
- Readable
- Comprehensible
- Elegant
Based on all of these definitions, is my code clean?Based on all of these definitions, is your code clean?
To clean it up, we’ll focus on these things:
- overall design of the class
- names
- functions
- formatting
- comments
Meaningful names
- Use Intention-Revealing Names
Functions
- Small
- Do one thing
Comments
- Comments do not make up for bad code
Formatting
- The Purpose of Formatting
- The Newspaper Metaphor
- Vertical Openness Between Concepts
- Vertical Density
- Vertical Distance
- Variable Declarations.
- Variables should be declared as close to their usage as possible.
- Dependent Functions.
- Conceptual Affinity
- Vertical Ordering
- Horizontal Formatting
- Horizontal Openness and Density